How to Add and Subtract Days to Dates in Flutter
Adding and subtracting days to a specific date is not straightforward. Here, I am showing you how to add or minus days from a given date in Flutter.
We have to make use of the DateTime class for this calculation purpose. We should also use methods such as add or subtract with the Duration class to get the desired results.
For example, if you want to add 30 days to today’s date then you can code it as given below.
var thirtyDaysFromNow = DateTime.now().add(new Duration(days: 30));
If you want to subtract 30 days then you can write it as given below.
var thirtyDaysMinusNow = DateTime.now().subtract(new Duration(days: 30));
Following is the complete example where I show the current date, added date, and subtracted date together.
I used the intl flutter package to show dates in specific date format.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage());
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Date Add and Subtract Example',
),
),
body: Center(
child: Column(
children: [
Text(DateFormat("MM-dd-yyyy").format(DateTime.now())),
Text(DateFormat("MM-dd-yyyy")
.format(DateTime.now().add(const Duration(days: 30)))),
Text(DateFormat("MM-dd-yyyy")
.format(DateTime.now().subtract(const Duration(days: 30))))
],
)));
}
}
Following is the output.
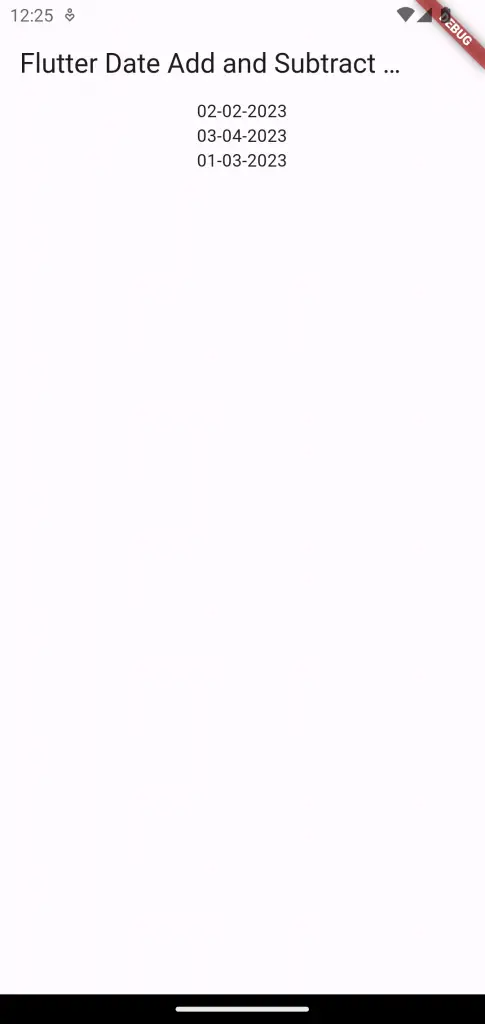
I hope this tutorial on Flutter date add and subtract will be helpful for you.
Thank you guys <3