Dynamically Hide and Unhide Widgets in Flutter
We know, a flutter app is composed of numerous widgets. In some scenarios, we may want to hide and show any specific widget depending on certain situations. This can be done easily using the Visibility class.
The widget which is to be hidden should be the child of the Visibility widget. The visibility is managed by the visible property of the Visibility class. Using state management properly makes visibility changes dynamic.
In the following example, there are two items, a Container, and an ElevatedButton. The Container is the child of the Visibility class. We used a stateful widget here to manage the hiding and showing of the container easily.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Hide and Show Widgets in Flutter',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatefulWidget {
FlutterExample({Key key}) : super(key: key);
@override
_FlutterExampleState createState() => _FlutterExampleState();
}
class _FlutterExampleState extends State<FlutterExample> {
var isVisible = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Hide / UnHide Widgets'),
),
body: Center(
child: Column(children: [
Visibility(visible: isVisible,
child:Container(
margin: const EdgeInsets.all(10.0),
color: Colors.amber,
width: 200.0,
height: 200.0,
),),
ElevatedButton(onPressed: () {
setState(() {
isVisible = !isVisible;
});
},
child: Text(' Elevated Button'))],)
),
);
}
}
Initially, the container is visible. When the button is pressed, the container disappears. The container is shown again as we press the button again. See the output of this Flutter example below.
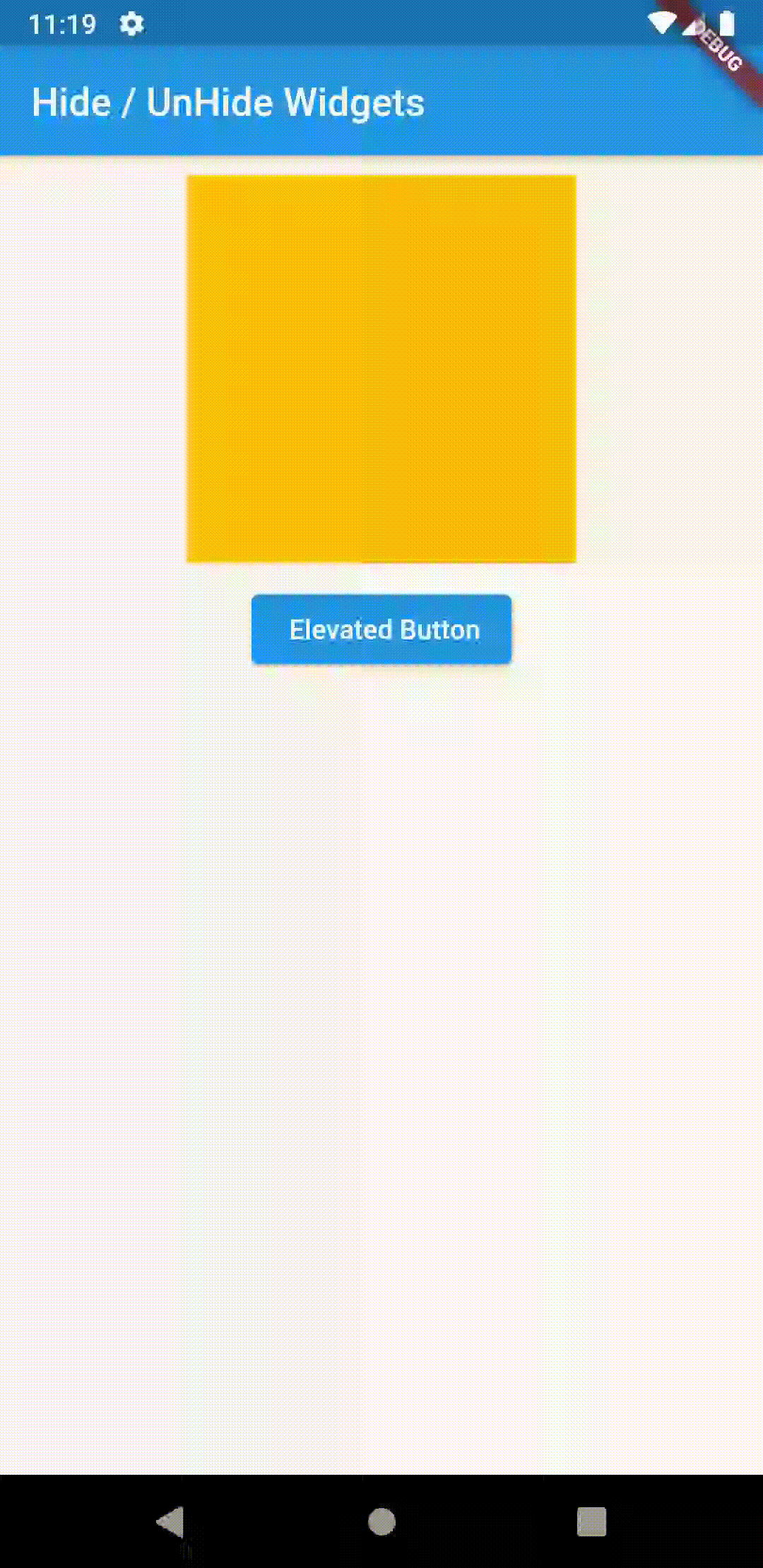
This is how you hide and unhide widgets in flutter. I hope this flutter tutorial will be helpful for you.