How to Dynamically Disable and Enable Button in Flutter
Sometimes, we may need to disable and enable buttons in our apps. In this flutter example, I am showing you how to enable and disable elevated button.
The ElevatedButton is enabled by default. But you can easily disable it by passing null to the onPressed property. See the snippet below.
ElevatedButton(onPressed:null,
child: Text('Disabled Button!'),),
In practical scenarios, you always wanted to enable and disable the button dynamically. This can be done using the stateful widget.
In the following example, I have two buttons. The top button will be either disabled or enabled by pressing the bottom button. We use a variable named status to change the value of onPressed property when the bottom button is pressed. See the complete flutter example to disable and enable the elevated button.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Enable and Disable Button in Flutter',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatefulWidget {
FlutterExample({Key key}) : super(key: key);
@override
_FlutterExampleState createState() => _FlutterExampleState();
}
class _FlutterExampleState extends State<FlutterExample> {
var status = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Enable / Disable Button'),
),
body: Center(
child: Column(children: [
ElevatedButton(onPressed: status?(){}: null,
child: Text('Check this Button!'),),
ElevatedButton(onPressed: () {
setState(() {
status = !status;
});
},
child: Text(' Disable / Enable'))],)
),
);
}
}
Following is the output of this Flutter tutorial.
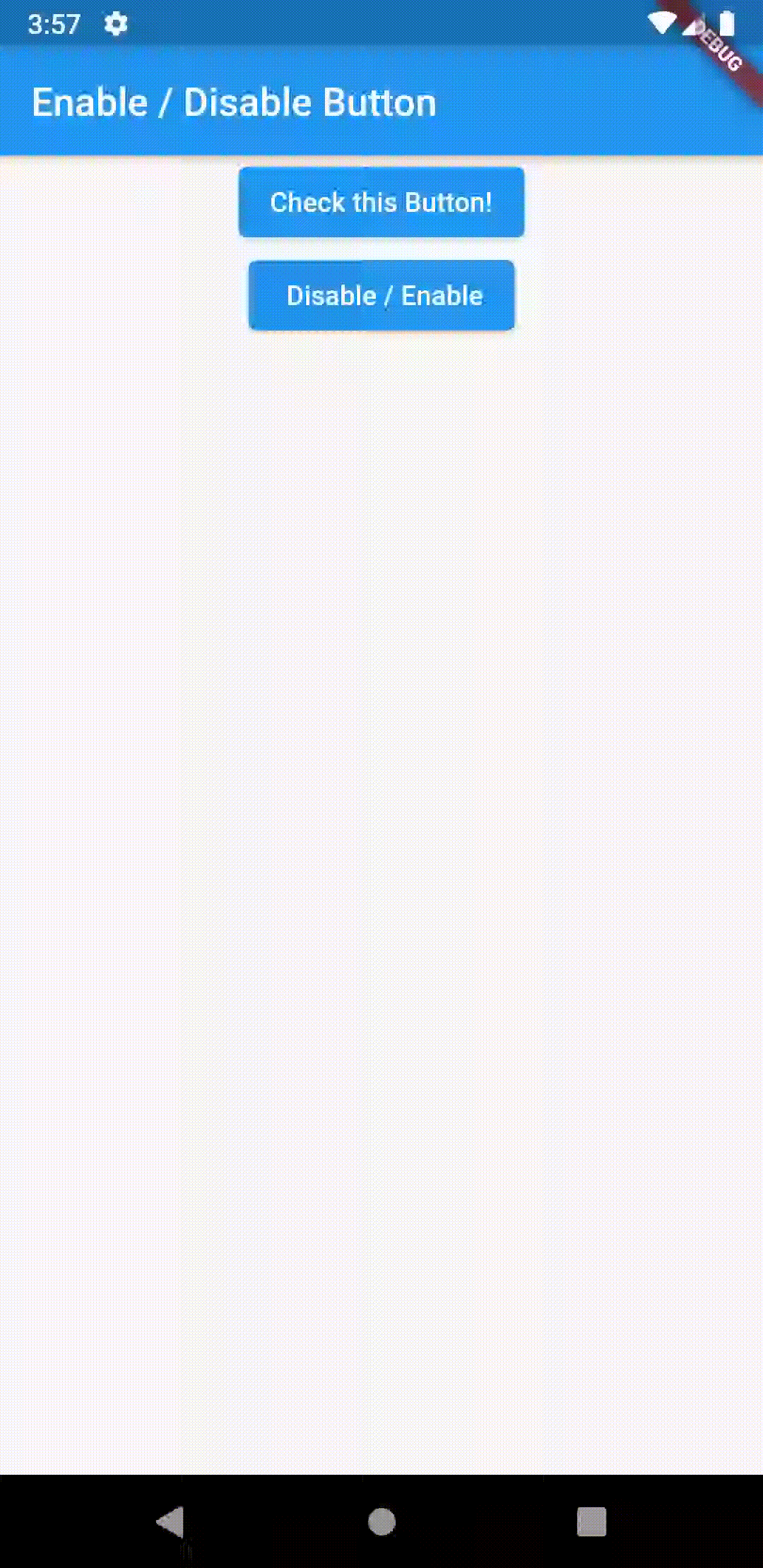
I hope you will find this tutorial helpful.