How to Double Underline Text in Flutter
There’s already a blog post on how to underline a text in Flutter. In this Flutter tutorial, let’s check how to underline text with two lines.
The TextStyle class is used to style Text Widget in Flutter. Using decoration property and TextDecoration class we can underline a text with a single line. You can use decorationStyle property and TextDecorationStyle class to make the underline double. See the code snippet given below.
Text(
'Double Underline',
style: TextStyle(
color: Colors.black
decoration: TextDecoration.underline,
decorationStyle: TextDecorationStyle.double,
),
)
If you want only specific text to be underlined with double lines then prefer RichText widget over Text Widget.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Double Underlined Text Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
const FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Flutter Double Underline Text')),
body: Center(
child: RichText(
text: TextSpan(
text: 'Here is ',
style: TextStyle(fontSize: 30, color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'Double',
style: TextStyle(
decoration: TextDecoration.underline,
decorationStyle: TextDecorationStyle.double,
)),
TextSpan(text: ' underlined text!'),
],
),
)));
}
}
Following is the output.
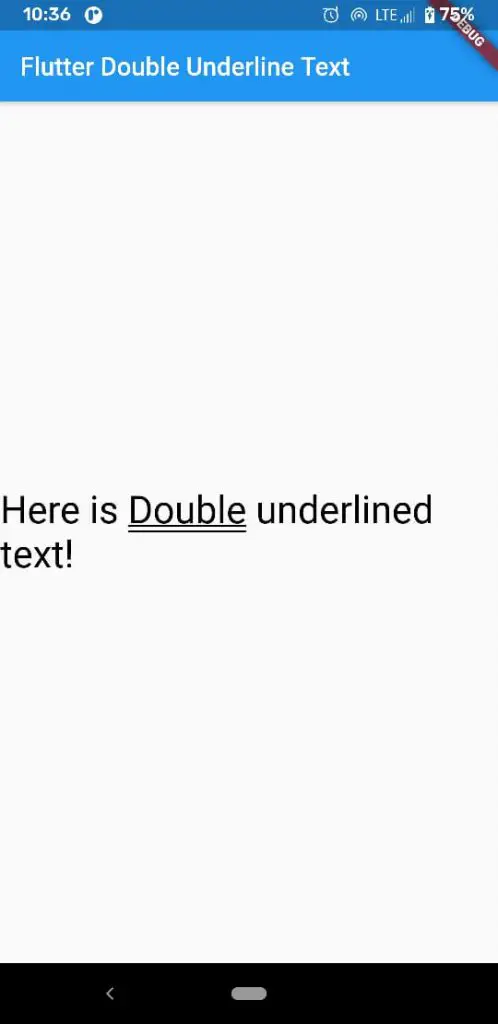
I hope this Flutter tutorial will be helpful for you.