How to show Linear Progress Indicator in Flutter
Progress Indicators have their own importance in mobile app UI. A progress indicator lets the user know that something is happening behind the screen. In this blog post, I will explain how to add a horizontal or linear progress indicator in Flutter.
The LinearProgressIndicator widget is used to display a horizontal progress indicator with material design in Flutter. See the code snippet given below.
LinearProgressIndicator(
backgroundColor: Colors.red,
color: Colors.yellow,
)
You will get the following output of linear progress indicator with yellow color and red color background.
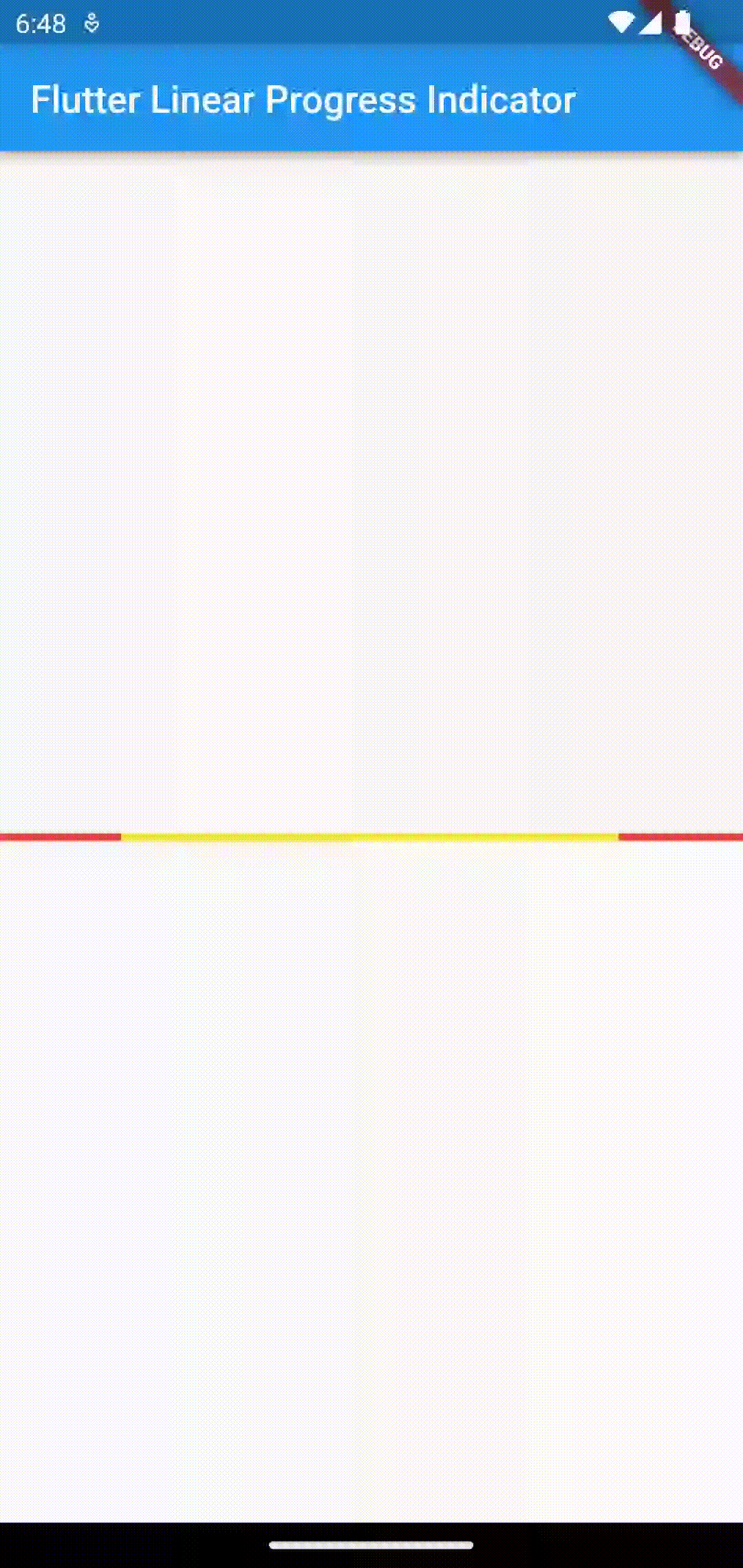
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Linear Progress Indicator'),
),
body: const Center(
child: LinearProgressIndicator(
backgroundColor: Colors.red,
color: Colors.yellow,
)));
}
}
Similarly, you can also add a circular progress indicator using CircularProgressIndicator class.
I hope this short Flutter tutorial on determinate linear progress indicator in Flutter is helpful for you.
One Comment